The Newsroom → Announcements → Jun 2nd, 2017
Partyline
Partyline
A neat little Laravel package that lets you dry up your console commands.
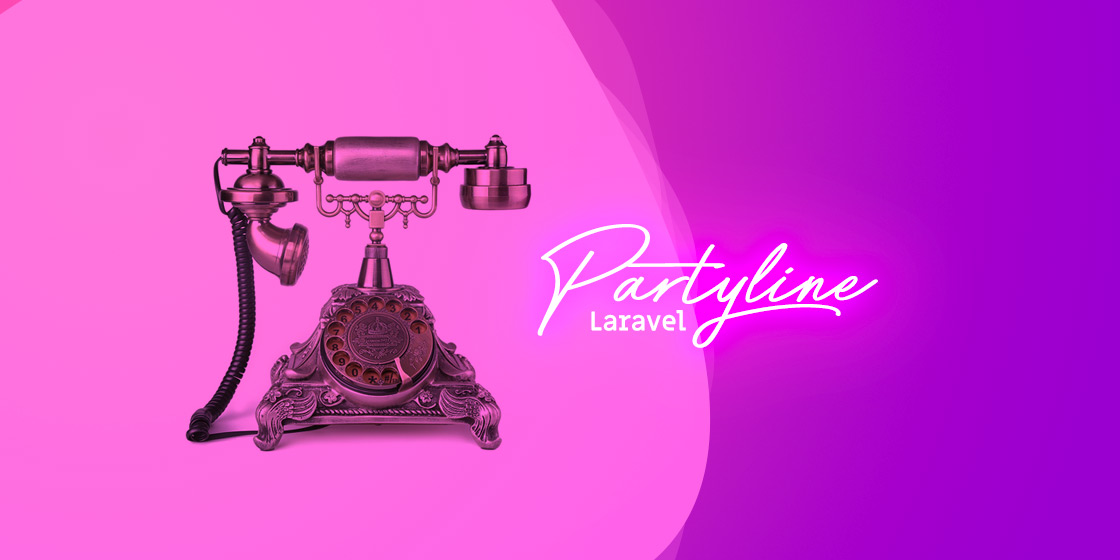
Partyline is a new Laravel package I developed to supercharge Statamic's command line (Artisan Console) integration.
Partyline allows you to push to the console from OUTSIDE your Command class.
Statamic has a number of features that have a browser and command line interface, like setting configs, generating image manipulation presets, or updating your search index.
Let's use updating the search index as an example. You can trigger an index update from the control panel, by hitting a URL, or or running php please search:update
. Behind the scenes we run Search::update()
and the deed is done.
On the command line side we have the ability to stream output to you as code is executed. We can show messages, progress bars, and so on. However, the mere act of sending feedback to the console would require duplicating the entire method and modifying it. Not very DRY. Not very awesome.
// Console command without Partylinepublic function handle(){ $this->line('Updating the index...'); Search::update(); // ¯\_(ツ)_/¯ $this->line('Surprise! It is finished!');}
Enter Partyline
With Partyline we can bake in console output feedback right into our single Search::update()
method. All Partyline code is ignored outside of the command line context.
// Our Search classclass Search { public function update() { Partyline::line('Updating the index...'); $entries = Entry::all(); $bar = Partyline::getOutput()->createProgressBar($entries->count()); foreach ($entries as $id => $entry) { $this->index->insert($id, $entry); $bar->advance(); } $bar->finish(); Partyline::line('All done!'); }}
// Console command *with* Partylineclass Command { public function handle() { Search::update(); }}
Partyline in action:
But wait...that's not SOLID.
If you're a design pattern purist, you may notice this goes against the single responsibility principle. Yes, the search updater in this example probably shouldn't be responsible for creating progress bars and outputting to the console, but which is worse? A little extra easy-to-read code in one place, or core application logic duplicated in two places, begging to be diverged and broken down over time?
Weigh the pros and cons. You might just fine the tradeoff to be well worth it. We did. If you find it to be useful, awesome! We'd love to hear about it.
Go Github it!
You can find Partyline on Github.